November 9, 2005
MSN Sandbox !!!
Guys check this out, Microsoft’s MSN sandbox (that’s how they called it), new MSN technologies, prototypes and many more. It’s really cool!! http://sandbox.msn.com/
November 7, 2005
System.Net.Mail
Seems to me that Microsoft has deprecated the System.Web.Mail, & replaced it with the System.Net.Mail. When I try to send an email using the System.Web.Mail it gives the message obsolete with a warning. Below is the code sample for sending simple SMTP emails with .NET 2.0.
public void SendEmail()
{
//.Net 1.0 System.Web.Mail -- Is now Depre
/*
//We can use the constructor as well; e.g.
/*MailMessage mail = new MailMessage("ludmal@test.com", "ludmal@tst.lk", "Test", "test");*/
MailMessage mail = new MailMessage();
mail.To = "ludmal@e-solutions.lk";
mail.From = "ludmal@test.com";
mail.Body = "Body Testing";
SmtpMail.SmtpServer = "mail";
SmtpMail.Send(mail);
*/
//.Net 2.0 System.Net.Mail
MailMessage mail = new MailMessage("ludmal@test.com", "ludmal@tst.lk", "Test", "test");
/*MailAddressCollection col = new MailAddressCollection();
MailAddress sendAdd = new MailAddress("ludmal@e-solutions.lk");
*/
System.Net.Mail.SmtpClient client = new SmtpClient("mysmtp");
client.Send(mail);
}
public void SendEmail()
{
//.Net 1.0 System.Web.Mail -- Is now Depre
/*
//We can use the constructor as well; e.g.
/*MailMessage mail = new MailMessage("ludmal@test.com", "ludmal@tst.lk", "Test", "test");*/
MailMessage mail = new MailMessage();
mail.To = "ludmal@e-solutions.lk";
mail.From = "ludmal@test.com";
mail.Body = "Body Testing";
SmtpMail.SmtpServer = "mail";
SmtpMail.Send(mail);
*/
//.Net 2.0 System.Net.Mail
MailMessage mail = new MailMessage("ludmal@test.com", "ludmal@tst.lk", "Test", "test");
/*MailAddressCollection col = new MailAddressCollection();
MailAddress sendAdd = new MailAddress("ludmal@e-solutions.lk");
*/
System.Net.Mail.SmtpClient client = new SmtpClient("mysmtp");
client.Send(mail);
}
November 4, 2005
Google's new RSS Reader
Google’s new RSS Feed reader. It gives you the complete power of ajax. Try it here http://www.google.com/reader/lens/ .
November 3, 2005
Socket Programming using .Net 2.0 System.Net.Socket
Socket is a communication mechanism where we use to connect client and the server. Basically the Server will listen to the client connections on specific port number. A client can connect to the Server by using the relevant hostname and the port number. Upon the successful client connection server will gets a new socket bound to a different port. It needs the original socket for listen to other client connections while communicating with the connected client. Below is the simple client /server example done using the .Net 2.0. Review the code & you will find it interesting. (I made it very simple to make you understand) You can modify the code to meet your needs.
Server Code:
class MyServer
{
//Listing to the client connections.
public void Listen()
{
byte[] ipArray = new byte[4];
ipArray[0] = 127;
ipArray[1] = 0;
ipArray[2] = 0;
ipArray[3] = 1;
IPAddress add = new IPAddress(ipArray);
//This is obselete in .Net 2.0
//TcpListener tcpListener = new TcpListener(10);
TcpListener tcpListener = new TcpListener(add, 10);
tcpListener.Start();
Socket socketForClient = tcpListener.AcceptSocket();
if (socketForClient.Connected)
{
Util.Out("client connected..");
NetworkStream netStream = new NetworkStream(socketForClient);
StreamWriter writer = new StreamWriter(netStream);
StreamReader reader = new StreamReader(netStream);
string str = "Message from Server : " + DateTime.Now.ToLongDateString();
writer.WriteLine(str);
Util.Out("What meessage do you want to send to client?");
writer.WriteLine(Console.ReadLine());
writer.Flush();
str = reader.ReadLine();
Util.Out(str);
reader.Close();
writer.Close();
netStream.Close();
}
socketForClient.Close();
Util.Out("Exiting...");
}
}
Client Code :
public class MyClient
{
//Connects to the server
public void Connect()
{
TcpClient socketForServer = null;
try
{
socketForServer = new TcpClient("localhost", 10);
Util.Out("Connected to the Server");
}
catch
{
Util.Out("Failed to connecto localhost");
Util.Out("Exiting..");
return;
}
NetworkStream netstream = socketForServer.GetStream();
StreamReader reader = new StreamReader(netstream);
StreamWriter writer = new StreamWriter(netstream);
try
{
string output1, output2;
output1 = reader.ReadLine();
output2 = reader.ReadLine();
Util.Out(output1);
Util.Out(output2);
writer.Flush();
reader.Close();
}
catch
{
Util.Out("Exception Occured! Exiting...");
}
netstream.Close();
}
}
This is small helper class…
public class Util
{
public static void Out(string s)
{
Console.WriteLine(s);
}
}
This is a simple console application. You can create two console application one for the server & one for the client. Run the server first then client app. If you want you can create nice windows applications using this two simple classes to communicate over network.
Server Code:
class MyServer
{
//Listing to the client connections.
public void Listen()
{
byte[] ipArray = new byte[4];
ipArray[0] = 127;
ipArray[1] = 0;
ipArray[2] = 0;
ipArray[3] = 1;
IPAddress add = new IPAddress(ipArray);
//This is obselete in .Net 2.0
//TcpListener tcpListener = new TcpListener(10);
TcpListener tcpListener = new TcpListener(add, 10);
tcpListener.Start();
Socket socketForClient = tcpListener.AcceptSocket();
if (socketForClient.Connected)
{
Util.Out("client connected..");
NetworkStream netStream = new NetworkStream(socketForClient);
StreamWriter writer = new StreamWriter(netStream);
StreamReader reader = new StreamReader(netStream);
string str = "Message from Server : " + DateTime.Now.ToLongDateString();
writer.WriteLine(str);
Util.Out("What meessage do you want to send to client?");
writer.WriteLine(Console.ReadLine());
writer.Flush();
str = reader.ReadLine();
Util.Out(str);
reader.Close();
writer.Close();
netStream.Close();
}
socketForClient.Close();
Util.Out("Exiting...");
}
}
Client Code :
public class MyClient
{
//Connects to the server
public void Connect()
{
TcpClient socketForServer = null;
try
{
socketForServer = new TcpClient("localhost", 10);
Util.Out("Connected to the Server");
}
catch
{
Util.Out("Failed to connecto localhost");
Util.Out("Exiting..");
return;
}
NetworkStream netstream = socketForServer.GetStream();
StreamReader reader = new StreamReader(netstream);
StreamWriter writer = new StreamWriter(netstream);
try
{
string output1, output2;
output1 = reader.ReadLine();
output2 = reader.ReadLine();
Util.Out(output1);
Util.Out(output2);
writer.Flush();
reader.Close();
}
catch
{
Util.Out("Exception Occured! Exiting...");
}
netstream.Close();
}
}
This is small helper class…
public class Util
{
public static void Out(string s)
{
Console.WriteLine(s);
}
}
This is a simple console application. You can create two console application one for the server & one for the client. Run the server first then client app. If you want you can create nice windows applications using this two simple classes to communicate over network.
November 2, 2005
C# 2.0 : String comparison
While i was reading some of the msdn blogs i found a nice article about string comparison in C# 2.0. Just have a look and it might be helpful to you. http://blogs.msdn.com/abhinaba/archive/2005/11/02/488041.aspx
Class diagrams in VS 2005
One of the loving features of mine in VS 2005 is class diagrams. The ability to draw class diagrams and the same time it will generate the code for us and vice-versa. Once you done the development you can simply generate the class diagram by right clicking on the Project > View Class Diagram. If you want to export the diagram as an image, right click on the diagram and select Export Diagram as Image. Below is the sample diagram I created using VS 2005
.
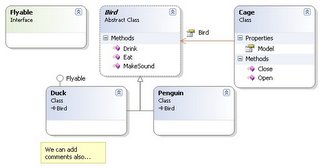
Subscribe to:
Posts (Atom)